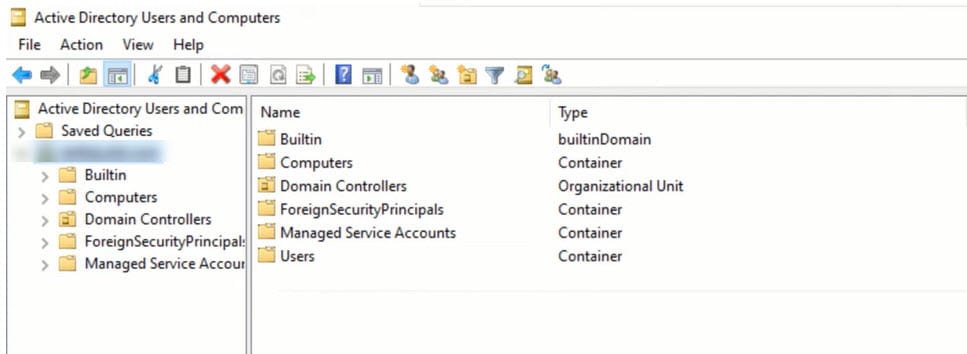
PowerShell provides a powerful way to retrieve information about user accounts and computer activity in your organization. The cmdlets discussed in this article can be used to retrieve information about the last logon date of a user, last contact of a computer, never logged on users, and users that logged in more than a year ago.
We’ll explore how to retrieve information about user accounts and computer activity using PowerShell. This information can be useful for auditing purposes, as well as for managing user accounts and computer resources.
Getting the Last Logon Date of Users:
To retrieve the last logon date users, you can use the following PowerShell cmdlet:
Import-Module ActiveDirectory
Get-ADUser -Filter * -Properties LastLogonDate | Select-Object -Property Name,LastLogonDate | Sort-Object -Property LastLogonDate -Descending
This cmdlet retrieves information about all user accounts in the Active Directory, including the last logon date. The output is sorted in descending order by the last logon date, so the most recent logons are displayed first.
Retrieving the Last Logon Date for a Specific User:
Here is an example code that demonstrates how to retrieve the last logon date for a specific user in Active Directory.
Import-Module ActiveDirectory
$User = Get-ADUser -Identity "Username" -Properties "LastLogonDate"
$User | Select-Object Name,LastLogonDate
In this code, the Get-ADUser
cmdlet is used to retrieve a specific user with the -Identity
parameter. The -Properties
parameter is used to specify that the LastLogonDate
property should be included in the results. The Select-Object
cmdlet is used to filter the output to only show the Name
and LastLogonDate
properties.
Replace Username
with the sAMAccountName of the desired user.
Finding Never Logged-On Users:
To find users who have never logged on, you can use the following PowerShell cmdlet:
Get-ADUser -Filter {Enabled -eq $True} -Properties LastLogonDate | Where-Object {$_.LastLogonDate -eq $Null} | Select-Object -Property Name
This cmdlet retrieves information about all enabled user accounts in the Active Directory and filters for accounts where the last logon date is equal to $Null, indicating that the user has never logged on.
Finding Users Who Logged In More Than a Year Ago:
To find users who logged in more than a year ago, you can use the following PowerShell cmdlet:
$CutOff = (Get-Date).AddDays(-365)
Get-ADUser -Filter * -Properties LastLogonDate | Where-Object {$_.LastLogonDate -lt $CutOff} | Select-Object -Property Name,LastLogonDate | Sort-Object -Property LastLogonDate -Descending
This cmdlet retrieves information about all user accounts in the Active Directory and filters for accounts where the last logon date is less than the current date minus 365 days, indicating that the user logged in more than a year ago. The output is sorted in descending order by the last logon date.
To retrieve information for a different time period, you can simply replace the value of “-365” in the scripts with your desired number. For example, to retrieve information for the last 3 years, replace “-365” with “-1095”.
Retrieving User Password Expiration Date for AD Users:
You can retrieve the password expiration date for users in Active Directory.
Import-Module ActiveDirectory
Get-ADUser -Filter * -Properties "msDS-UserPasswordExpiryTimeComputed" | Select-Object Name,"msDS-UserPasswordExpiryTimeComputed"
This script imports the ActiveDirectory module and retrieves all users in the directory. The -Properties
parameter is used to specify that the msDS-UserPasswordExpiryTimeComputed
property should be included in the results. The Select-Object
cmdlet is used to filter the output to only show the Name
and msDS-UserPasswordExpiryTimeComputed
properties.
This script will give you the password expiration date for all users in your Active Directory. If you only want to retrieve the password expiration date for a specific user, you can replace the -Filter *
parameter with the distinguished name (DN) or the sAMAccountName of the desired user.
Retrieving the Password Expiration Date for a Specific User:
Here is an example code that demonstrates how to retrieve the password expiration date for a specific user in Active Directory.
Import-Module ActiveDirectory
$User = Get-ADUser -Identity "sAMAccountName" -Properties "msDS-UserPasswordExpiryTimeComputed"
$User | Select-Object Name,"msDS-UserPasswordExpiryTimeComputed"
In this code, the Get-ADUser
cmdlet is used to retrieve a specific user with the -Identity
parameter. The -Properties
parameter is used to specify that the msDS-UserPasswordExpiryTimeComputed
property should be included in the results. The Select-Object
cmdlet is used to filter the output to only show the Name
and msDS-UserPasswordExpiryTimeComputed
properties.
Replace sAMAccountName
with the sAMAccountName of the desired user.
Retrieving the Last Password Change Date for Users:
You can retrieve the last password change date users in Active Directory using the following PowerShell script.
Import-Module ActiveDirectory
Get-ADUser -Filter * -Properties PasswordLastSet | Select-Object Name,PasswordLastSet
Retrieving the Last Password Change for a Specific User:
To retrieve the last password change date for a specific user in Active Directory, you can use the following script.
$User = Get-ADUser -Identity "Username" -Properties "PasswordLastSet"
$User | Select-Object Name,PasswordLastSet
In this script, the Get-ADUser cmdlet is used to retrieve a specific user with the -Identity parameter. The -Properties parameter is used to specify that the PasswordLastSet property should be included in the results. The Select-Object cmdlet is used to filter the output to only show the Name and PasswordLastSet properties.
Replace Username
with the sAMAccountName of the desired user.
Getting Last Contact Date of a Computers:
To retrieve the last contact of computers, you can use the following PowerShell script.
Get-ADComputer -Filter * -Properties LastLogonDate | Select-Object -Property Name,LastLogonDate | Sort-Object -Property LastLogonDate -Descending
This cmdlet retrieves information about all computers in the Active Directory, including the last logon date. The output is sorted in descending order by the last logon date, so the most recent contacts are displayed first.
Retrieve the Last contact Date for a Specific Computer:
Here is an example code that demonstrates how to retrieve the last contact date for a specific computer in Active Directory.
Import-Module ActiveDirectory
$Computer = Get-ADComputer -Identity "ComputerName" -Properties "LastLogonDate"
$Computer | Select-Object Name,LastLogonDate
In this code, the Get-ADComputer
cmdlet is used to retrieve a specific computer with the -Identity
parameter. The -Properties
parameter is used to specify that the LastLogonDate
property should be included in the results. The Select-Object
cmdlet is used to filter the output to only show the Name
and LastLogonDate
properties.
Replace ComputerName
with the name of the desired computer.