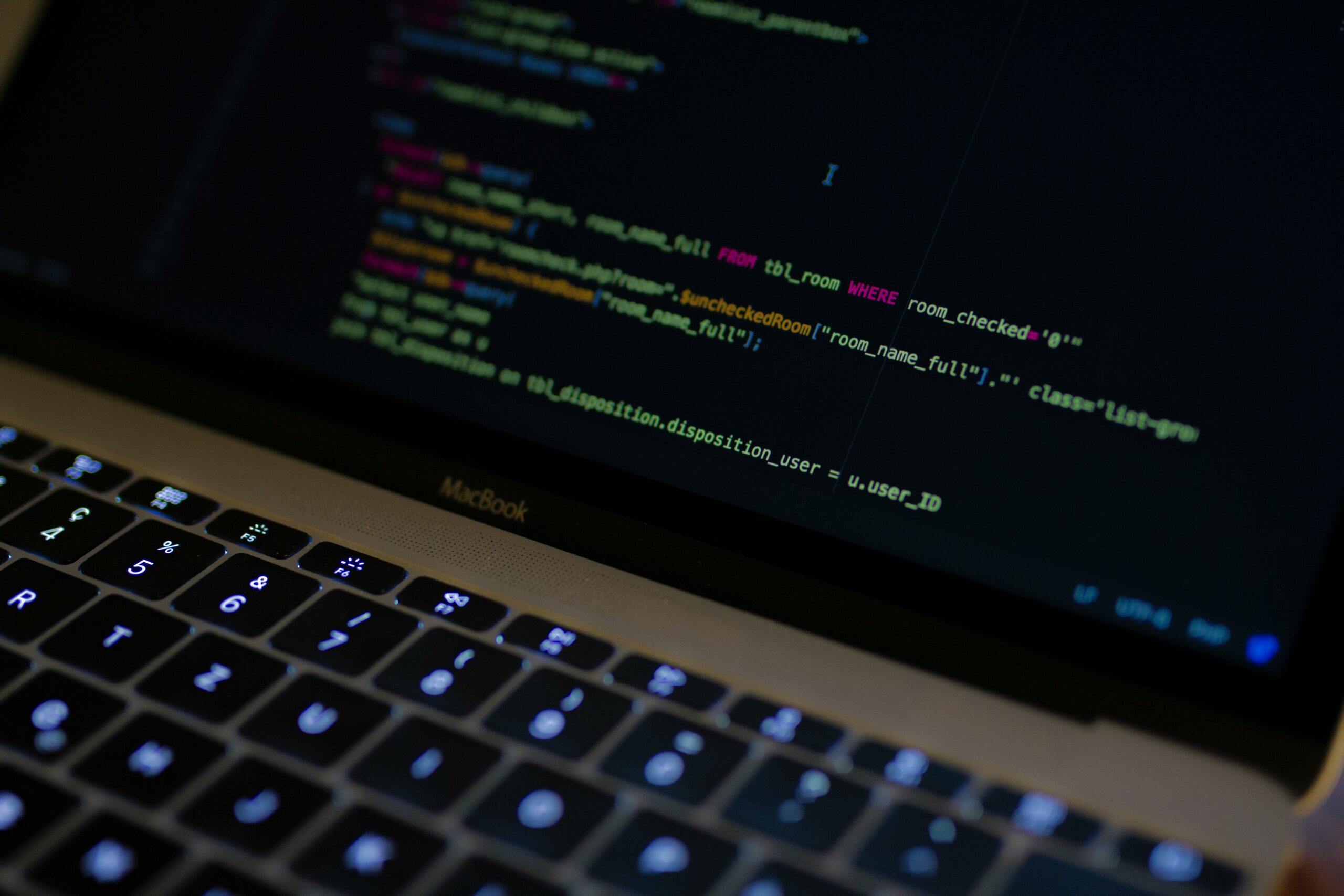
1. SELECT * FROM table_name:
This query will retrieve all of the data from a specific table.
SELECT * FROM table_name;
2. SELECT column_name FROM table_name:
This query will retrieve a specific column from a table.
SELECT column_name FROM table_name;
3. SELECT * FROM table_name WHERE condition:
This query will retrieve all of the data from a table where a certain condition is met. For example, “SELECT * FROM users WHERE age > 30” will retrieve all rows from the “users” table where the age column is greater than 30.
SELECT * FROM table_name WHERE condition;
4. INSERT INTO table_name (column_1, column_2) VALUES (value_1, value_2):
This query will insert a new row into a table, with the specified values for each column.
INSERT INTO table_name (column_1, column_2) VALUES (value_1, value_2);
5. UPDATE table_name SET column_name = new_value WHERE condition:
This query will update the value of a specific column in a table, where a certain condition is met.
UPDATE table_name SET column_name = new_value WHERE condition;
6. DELETE FROM table_name WHERE condition:
This query will delete rows from a table where a certain condition is met.
DELETE FROM table_name WHERE condition;
7. CREATE TABLE table_name (column_1 datatype, column_2 datatype):
This query will create a new table with the specified column names and data types.
CREATE TABLE table_name (column_1 datatype, column_2 datatype);
8. ALTER TABLE table_name ADD column_name datatype:
This query will add a new column to an existing table.
ALTER TABLE table_name ADD column_name datatype;
9. DROP TABLE table_name:
This query will delete a table from the database.
DROP TABLE table_name;
10. SELECT COUNT(*) FROM table_name:
This query will return the number of rows in a table.
SELECT COUNT(*) FROM table_name;
11. SELECT DISTINCT column_name FROM table_name:
This query will return only unique values from a specific column in a table.
SELECT DISTINCT column_name FROM table_name;
12. SELECT column_name1, column_name2 FROM table_name:
This query will retrieve multiple columns from a table.
SELECT column_name1, column_name2 FROM table_name;
13. SELECT column_name FROM table_name WHERE condition ORDER BY column_name:
This query will retrieve data from a table and sort the results by a specific column.
SELECT column_name FROM table_name WHERE condition ORDER BY column_name;
14. SELECT column_name FROM table_name LIMIT number:
This query will retrieve a specific number of rows from a table.
SELECT column_name FROM table_name LIMIT number;
15. SELECT * FROM table_name INNER JOIN table_name ON condition:
This query will retrieve data from two tables, using an inner join to match rows that meet the specified condition.
SELECT * FROM table_name INNER JOIN table_name ON condition;
16. SELECT * FROM table_name LEFT JOIN table_name ON condition:
This query will retrieve data from two tables, using a left join to include all rows from the first table and any matching rows from the second table.
SELECT * FROM table_name LEFT JOIN table_name ON condition;
17. SELECT * FROM table_name RIGHT JOIN table_name ON condition:
This query will retrieve data from two tables, using a right join to include all rows from the second table and any matching rows from the first table.
SELECT * FROM table_name RIGHT JOIN table_name ON condition;
18. SELECT column_name FROM table_name WHERE column_name IN (value1, value2, value3):
This query will retrieve data from a table where the value of a specific column matches any of the specified values.
SELECT column_name FROM table_name WHERE column_name IN (value1, value2, value3);
19. SELECT column_name FROM table_name WHERE column_name BETWEEN value1 AND value2:
This query will retrieve data from a table where the value of a specific column is between two specified values.
SELECT column_name FROM table_name WHERE column_name BETWEEN value1 AND value2;
20. SELECT column_name FROM table_name WHERE column_name LIKE pattern:
This query will retrieve data from a table where the value of a specific column matches a specified pattern. The pattern can include wildcards such as % (matches any number of characters) and _ (matches a single character).
SELECT column_name FROM table_name WHERE column_name LIKE pattern;